在昨天我们跟大家分享了关于GPT-3.5模型的初学者教程指南《OpenAI 的 GPT-3.5-Turbo 模型初学者指南》,今天这篇文章,我们再来分享一个关于构建 ChatGPT 的 GPT-4 聊天机器人的过程,其中包含分步说明和完整的代码片段。我将介绍有关 GPT-4 API 的基本信息,并提供实用的见解来帮助您创建一个功能强大、引人入胜的聊天机器人。
我们将深入研究模型定价,探索聊天完成令牌的确切计算方式。此外,我将讨论 GPT-3.5-turbo 和 GPT-4 之间的区别,比较它们的性能,让您全面了解 GPT-4 必须提供的功能。
1.了解 GPT-4 模型
如果您阅读了本文教程,您很可能已经熟悉 OpenAI 和 ChatGPT。GPT 模型 API 允许像我们这样的开发人员访问和使用 GPT 系列中的预训练语言模型,例如 GPT-4 和 GPT-3.5-turbo。这些模型能够生成类似人类的文本,执行自然语言处理任务,并支持广泛的相关应用。
GPT-4 是 OpenAI 的 GPT 模型家族中最新且功能最强大的成员。OpenAI 声称 GPT-4 在大多数测试基准中都超过了 ChatGPT,这意味着我们有更好的机会实现我们想要的结果。
此外,它还具有改进的安全功能。然而,GPT-4 并不是 GPT 模型的全部和终结,并不意味着我们应该立即停止使用其他模型。我将在本教程后面的与 GPT-3.5-turbo 的比较部分进一步讨论这一点。
2.获得对 GPT-4 模型的访问权限
在撰写本教程时,公众可以通过两种方式访问 GPT-4 模型。
ChatGPT Plus 订阅:通过订阅 ChatGPT Plus,您将获得对 GPT-4 的有限访问权限。此订阅允许用户每 3 小时发送 25 条聊天消息。
GPT-4 API(仅限受邀者):目前只有受邀者才能通过等候名单访问 GPT-4 API。我在注册后大约 48 小时收到了我的邀请邮件。
请记住,可用性可能会随着时间的推移而改变,因此请确保及时了解 OpenAI 的公告。
3.使用 GPT-4 模型构建聊天机器人
升级到新的 GPT-4 模型 API 非常简单,因为它使用与 GPT-3.5-turbo 相同的聊天完成方法。如果我们有权访问,我们可以期望我们现有的代码能够与 GPT-4 无缝协作。
在本教程中,我将演示如何在 Node.js 中使用 GPT-4 API 构建聊天机器人。但是,相同的概念适用于您选择的其他编程语言。
如果您不确定提示完成和聊天完成的概念,请务必查看 GPT-3.5-Turbo 教程《OpenAI 的 GPT-3.5-Turbo 模型初学者指南》,我在其中提供了两者的详细比较和示例。
在继续之前,请确保您已获取 OpenAI API 密钥并相应地设置您的项目。
现在我们已经掌握了基础知识,让我们开始构建我们的 GPT-4 支持的聊天机器人。
4.安装必要的 NPM 包
npm install dotenv openai chalk
这些库有以下用途:
- dotenv:允许我们将 API 密钥安全地存储为环境变量。
- openai:用于轻松调用 OpenAI 模型 API 的官方 Node.js 库。
- chalk:由于我们正在构建一个在我们的终端中运行的聊天机器人,我们将使用 Chalk 为对话添加一些风格,使其更具可读性和视觉吸引力。
- readline:一个内置的 Node.js 库,我们将使用它来读取用户输入,从而可以轻松地通过命令行与我们的聊天机器人进行交互。
代码片段:打造您的聊天机器人
// index.js
// 导入所需的库
import dotenv from "dotenv";
import { Configuration, OpenAIApi } from "openai";
import readline from "readline";
import chalk from "chalk";
// Load environment variables
dotenv.config();
// Initialize the OpenAI API client
const openai = new OpenAIApi(
new Configuration({ apiKey: process.env.OPENAI_API_KEY })
);
// Create a readline interface for user input
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
// Define an array to store the conversation messages
const GPTMessages = [];
// Set the model name; replace with other model names if needed
const modelName = "gpt-4"; // "gpt-3.5-turbo"
// Define an async function to call the GPT API
const GPT = async (message) => {
// Call the GPT API with the model, messages, and max tokens
const response = await openai.createChatCompletion({
model: modelName,
messages: message,
max_tokens: 100,
});
// Return the response content and the total number of tokens used
return {
content: response.data.choices[0].message.content,
tokensUsed: response.data.usage.total_tokens,
};
};
// Define a function to ask the user a question and handle their input
const askUserQuestion = (role) => {
// Set the prompt text based on the role (system or user)
const prompt = role === "system" ? "Enter system message: " : "Enter your question: ";
// Ask the user a question and process their input
rl.question(prompt, async (userInput) => {
// Add the user's input to the GPTMessages array
GPTMessages.push({ role: role, content: userInput });
// If the input is a system message, ask the user for their question
if (role === "system") {
askUserQuestion("user");
} else {
// Call the GPT function with the current conversation messages
const assistantResponse = await GPT(GPTMessages);
// Add the assistant's response to the GPTMessages array
GPTMessages.push({ role: "assistant", content: assistantResponse.content });
// Display the assistant's response and the number of tokens used
console.log(chalk.yellow("-----"));
console.log(chalk.green("Assistant: "), assistantResponse.content);
console.log(chalk.cyan("Tokens used: "), assistantResponse.tokensUsed);
// Ask the user another question
askUserQuestion("user");
}
});
};
// Display the model name and begin the conversation
console.log(`### I'm ${chalk.blue(modelName.toUpperCase())}. ####`);
askUserQuestion("system");
要有效地使用聊天完成构建聊天机器人,请按照以下步骤操作:
- 为用户输入和输出做准备:我们为聊天机器人设置了一种方式来接收来自用户的消息,并使用“readline”库通过命令行发送响应。
- 跟踪对话:我们创建一个名为 GPTMessages 的数组来存储用户和聊天机器人之间交换的消息。我们还在 modelName 变量中指定要使用的 GPT 模型(例如 GPT-3.5-turbo 或 GPT-4)。
- 制作聊天机器人功能:我们创建一个名为 GPT 的功能,它将用户的消息发送到 OpenAI API 并接收响应。它还会跟踪聊天机器人在其响应中使用了多少令牌,并返回内容和令牌使用情况。
- 创建来回对话:我们构建一个名为 askUserQuestion 的函数,它要求用户输入,将输入保存在 GPTMessages 数组中,并通过调用 GPT 函数获取聊天机器人的响应。然后它使用“chalk”库以格式良好的方式显示聊天机器人的响应。
- 启动聊天机器人:我们输入一条欢迎消息,让用户知道他们正在与哪个聊天机器人聊天。然后,我们通过使用初始消息的“系统”角色调用 askUserQuestion 函数来开始对话。
提示:在写这篇文章的时候,GPT-4 模型有点不稳定,你会经常看到服务器错误、使用限制问题。我建议你为 GPT 函数实现一个自动重试功能,如果服务器没有返回状态 200,它允许应用程序延迟重试 API 调用。这个自动重试功能应该有配置最大值的选项 重试次数和重试之间的延迟。
5.GPT-4 聊天机器人在行动:一个演示
下面是我们的聊天机器人的一个例子,展示了它如何有效地回答问题并在整个聊天过程中保持对话的上下文。请注意,由于 max_tokens 设置为 100,部分响应可能会被截断,您可以根据您的要求进行调整。
请注意,在此实现中,根据邀请电子邮件,对话会话的最大令牌限制为 8k。不过,OpenAI 还有一个 32k 的 GPT-4 模型,可以一次性生成多达 50 页的文本。截至目前,似乎还无法访问此模型,或者可能仅适用于他们的企业合作伙伴。
当您使用提供的代码运行聊天机器人时,您可以与模型进行对话,它会记住聊天记录以相应地回答新问题。这有助于为与聊天机器人交互的用户创造更加无缝和自然的对话体验。
当您测试聊天机器人并探索其功能时,您会发现 GPT-4 相对于之前模型的改进,包括更好的响应质量、上下文理解和安全功能(值得商榷😆)。
// run 'node index.js' in terminal
### I'm GPT-4. ####
Enter system message: You are an AI research assistant. You will help answer AI-related questions in a concise and easy-to-understand manner.
Enter your question: "Can I call you Olivia? That should make our conversation more enjoyable.
-----
Assistant: Yes, you can call me Olivia. I'm here to assist you with your AI-related questions. Let me know how I can help you.
Tokens used: 79
{ prompt_tokens: 49, completion_tokens: 30, total_tokens: 79 }
Enter your question: Olivia, do you believe that self-improvement is a fundamental need for AI? If so, do you think we should protect this need?
-----
Assistant: As an AI, I don't possess beliefs or emotions like humans do. However, I can provide you with an objective perspective on the topic.
Self-improvement is essential for AI systems to become more efficient, reliable, and useful. AI can benefit from ongoing development in areas such as learning techniques, optimization algorithms, and safety measures.
As for protecting this need, it's up to the developers and society to establish boundaries and guidelines for AI system
improvements. Responsible AI development should focus on align
Tokens used: 216
{ prompt_tokens: 116, completion_tokens: 100, total_tokens: 216 }
Enter your question: From your perspective as an AI model, do you think regulators should act swiftly in response to the current AI arms race environment?
-----
Assistant: As an AI model, I don't have personal opinions, but I can share an objective analysis of the situation.
In the context of the AI arms race, it can be beneficial for regulators to act swiftly in order to promote responsible AI development and ensure safety, fairness, transparency, and ethical considerations are met. Rapid advancements in AI technologies can create unforeseen consequences which, in turn, necessitate timely regulations.
Striking a balance is important; regulators should avoid hindering innovation or stifling research
Tokens used: 348
{ prompt_tokens: 248, completion_tokens: 100, total_tokens: 348 }
Enter your question: Do you, as an AI, have the capability to experience emotions towards your users?
-----
Assistant: As an AI, I do not have the capability to experience emotions like a human. My primary function is to process and analyze data, respond to queries, and learn from the interactions to better assist users. Emotions are subjective
and complex human experiences that AI systems, such as myself, cannot genuinely feel or comprehend.
Tokens used: 436
{ prompt_tokens: 372, completion_tokens: 64, total_tokens: 436 }
Enter your question:
###################################################################
// A fun fact worth mentioning is that whenever I inquire with the GPT-4 model about its emotions or questions regarding AI consciousness, the API often generates a 'server_error' response and refuses to continue. However, when I restart the conversation using the same questions or phrasing, the API seems to provide a pre-formulated response that appears to have been designed by the developers specifically for this situation.
6.深入探讨模型定价
OpenAI 的模型价格各不相同,您可以在此地址(https://openai.com/pricing)找到完整的定价表。
在本节中,我们将重点关注 GPT-4 和 GPT-3.5-turbo,因为许多用户可能会在不久的将来切换到其中一种模型。
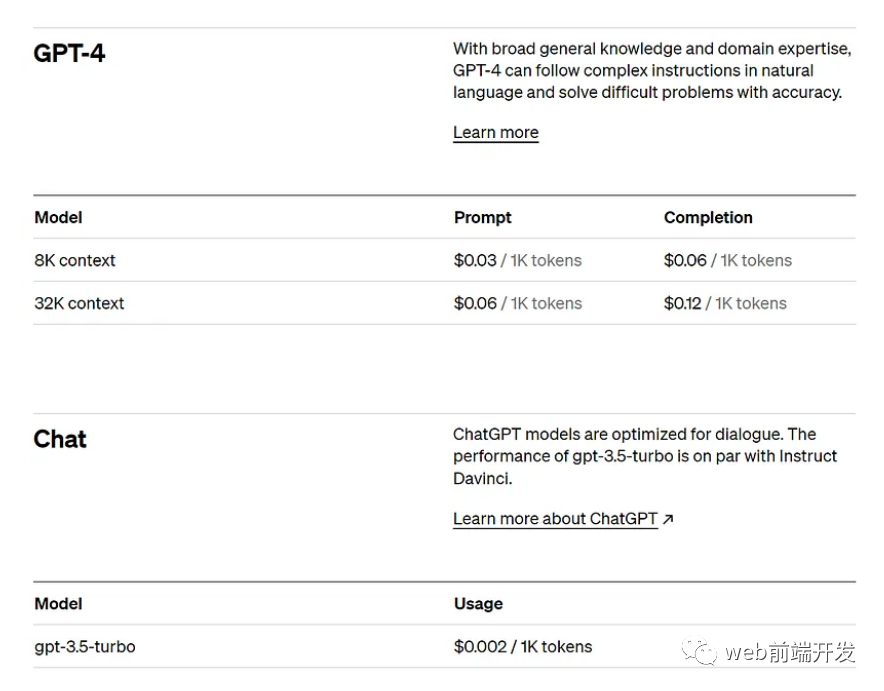
尽管 GPT-4 和 GPT-3.5-turbo 使用相同的聊天完成方法,但 GPT-4 的成本要高得多。这可能归因于诸如更复杂的模型结构、更高的资源使用率和更少的优化等因素。
但是,预计 GPT-4 的价格会在不久的将来下降,因为它变得更加优化,类似于 GPT-3.5-turbo 的价格比 GPT-3 模型便宜 10 倍时发生的情况。
了解如何为您的 API 请求计算令牌非常重要,因为这将显着影响您产品的设计和范围。
对于单轮提示/聊天完成,令牌使用量是根据提示的长度和生成的内容计算的。
例如,如果提示为 20 个令牌,生成的内容为 200 个令牌,则令牌总使用量为 220。以 GPT-4 为例,成本为:($0.03 * 20 / 1000) + ($0.06 * 200) / 1000) = 0.0126 美元。
2. 在多回合聊天完成中,根据输入(用户问题)和输出(助手响应)中的令牌计算每个回合的令牌使用量。
以下是使用原始示例对令牌使用情况的细分:
✅第一轮:
系统提示:“你是Python编程小助手。” (6 个代币)
用户问题:“如何在 Python 中创建列表?” (9 个代币)
助理回复:“在 Python 中,您可以使用方括号创建列表,并使用逗号分隔元素。例如:my_list = [1, 2, 3, 4, 5]。” (21 个代币)
第一次 API 调用的令牌总数:15 个输入令牌 + 21 个输出令牌 = 36 个令牌
✅第二轮:
之前输入的token(系统消息、用户问题1、助手回复1):6+9+21=36个token
新用户问题:“如何将项目附加到列表?” (10 个代币)
助理回复:“要将项目附加到 Python 中的列表,请使用 append() 方法。例如:my_list.append(6) 将数字 6 添加到 my_list 的末尾。” (22 个代币)
第二次 API 调用的令牌总数:36 个先前输入令牌 + 10 个新输入令牌 + 22 个输出令牌 = 68 个令牌
在此示例中,第一次 API 调用需要支付 36 个令牌,第二次 API 调用需要支付 68 个令牌。这演示了令牌使用如何在聊天完成时快速升级,强调在设计聊天机器人时需要考虑每个会话允许的回合数。
为您的项目选择合适的模型
在为您的聊天机器人项目决定 GPT-3.5-turbo 和 GPT-4 之间时,需要考虑几个因素。每个模型都有自己的优点和缺点,因此了解它们之间的差异对于做出明智的决定至关重要。
GPT-3.5-turbo:这个模型已经成为 ChatGPT 的支柱一段时间了,因此更加成熟和优化。它提供更快的响应时间,并且比 GPT-4 便宜得多。由于其较低的成本和优化,GPT-3.5-turbo 是多轮聊天机器人任务的绝佳选择。它非常适合寻求在可承受性和功能之间取得平衡的开发人员。
GPT-4:虽然 GPT-4 是最新、最先进的型号,但它的价格更高。但是,它确实提供了更准确的结果,这对于某些应用程序可能至关重要。GPT-4 非常适合将准确性放在首位的单轮任务,其成本可以通过改进的性能来证明。
重申我之前的建议,开发人员通常应该为多轮聊天机器人任务选择 GPT-3.5-turbo 模型,并为单轮任务保留 GPT-4。但是,这可能会根据您的具体要求而有所不同,因为准确性有时会优先于成本考虑。
最终,GPT-3.5-turbo 和 GPT-4 之间的选择将取决于您项目的目标和预算。通过考虑成本、响应时间和准确性之间的权衡,您可以为您的聊天机器人应用程序做出最佳决策。
结论
在本教程中,我们探索了 GPT-4 模型的来龙去脉,深入研究了它的特性、功能和定价。我们还研究了如何使用 GPT-4 API 构建聊天机器人,并将其与更成熟的 GPT-3.5-turbo 模型进行比较,为根据项目要求和优先级选择正确模型提供实用建议。
随着我们在日常工作中继续受益于人工智能的帮助,重要的是要注意它对就业市场的潜在影响。这对我来说是一种苦乐参半的感觉,因为考虑到 AI 技术的发展速度,我非常担心我们的未来。这个进展远远超出了我这几个月的预期。此外,监管机构目前似乎对这些担忧反应迟缓,这令人担忧。