在当今这个社会,数据就是财富,数据就是金钱,一切都离不开数据,我们看到的一切图片,本质上都是数据,如何理解和处理这些图像数据是很大的难题,不过庆幸的是,在 python 中,已经有了非常丰富的扩展来帮助我们处理这些图片。
opencv
opencv 是一个非常流行的数据可视化图形库,它底层使用 c++进行开发,拥有非常高效的执行效率。
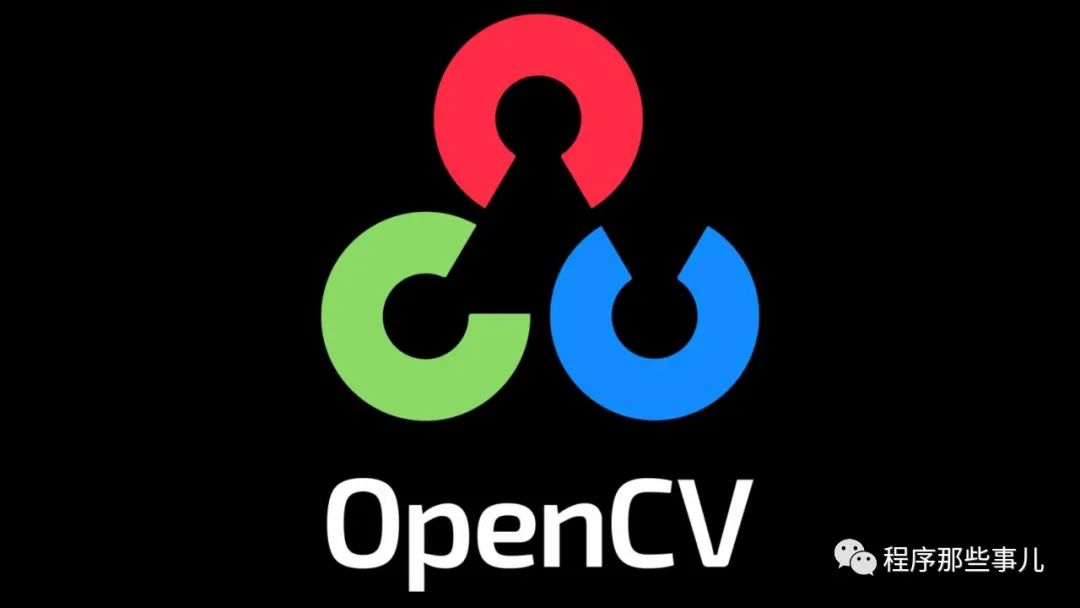
安装使用它非常简单。
pip install opencv-python
# import opencv
import cv2
# Read the image
image = cv2.imread('tesla.png')
# grayscale the image
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow('Original Image', image)
cv2.imshow('Grayscale Image', gray_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
Pillow
Pillow 是另一个非常流行的图像处理库,和 opencv 相比,它更加轻量级,虽然本身功能简单,但是它支持扩展,通过扩展可以执行非常强大的功能。
from PIL import Image
with Image.open("tesla.png") as im:
#show the original image
im.show("Original Image")
#convert into grayscale
grayscaleImg = im.convert("L")
#show the grayscale image
grayscaleImg.show()
Scikit
Scikit 是一个进行科学研究的图形处理库,旨在使用 Numpy 和 Scipy 库处理图像。它包括各种科学算法,例如分割、颜色空间操作、分析、形态学等。该库是使用 Python 和 C 编程语言编写的。它适用于所有流行的操作系统,例如 Linux、macOS 和 Windows。
from skimage import io
from skimage.color import rgb2gray
# way to load car image from file
car = io.imread('tesla.png')[:,:,:3]
#convert into grayscale
grayscale = rgb2gray(car)
#show the original
io.imshow(car)
io.show()
#show the grayscale
io.imshow(grayscale)
io.show()
Numpy
numpy 本身是一个计算库,它提供了广泛的数学特性,如数组、线性代数、基本统计运算、随机模拟、逻辑排序、搜索、形状操作等。
通过对图片的运算处理,可以实现图片的灰度化。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
#load the original image
img_rgb = mpimg.imread('tesla.png')[...,:3]
#show the original image
plt.imshow(img_rgb)
plt.show()
#convert the image into grayscale
img_gray = np.dot(img_rgb,[0.299, 0.587, 0.144])
#show the grayscale image
plt.imshow(img_gray, cmap=plt.get_cmap('gray'))
plt.show()
mahotas
Mahotas 是另一个可以执行各种图像处理操作的 Python 计算机视觉库。它是用 C++设计的,它包含许多提高图像处理速度的算法。此外,它使用 NumPy 数组在矩阵中使用图像。分水岭、凸点计算 hit & miss 卷积和 Sobel 边缘是该库中可用的主要功能。
import mahotas
from pylab import imshow, show
#read the image
img = mahotas.imread('tesla.png')
#show original image
imshow(img)
show()
img = img[:, :, 0]
grayscale = mahotas.overlay(img)
#show grayscale image
imshow(grayscale)
show()
SimpleITK
SimpleITK 是一个强大的图像配准和分割工具包。它是作为 ITK 工具包的扩展构建的,用于提供简化的界面。它支持不同的编程语言,例如 Python、R、C++、Java、C#、Ruby、TCL 和 Lua。
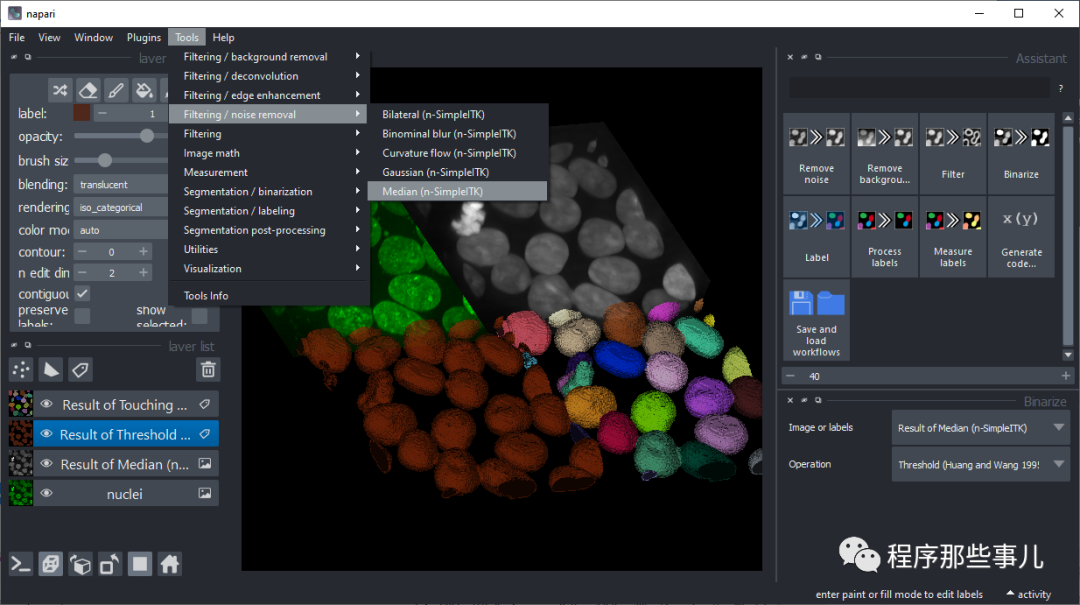
该库支持 2D、3D 和 4D 图像。与其他 Python 图像处理库和框架相比,该库的图像处理速度非常快。
import SimpleITK as sitk
import matplotlib.pyplot as plt
logo = sitk.ReadImage('tesla.png')
# GetArrayViewFromImage returns an immutable numpy array view to the data.
plt.imshow(sitk.GetArrayViewFromImage(logo))
plt.show()
Matplotlib
Matplotlib 是一个综合库,用于在 Python 中创建静态、动画和交互式可视化。Matplotlib 让简单的事情变得简单,让困难的事情成为可能。它可以配合 Numpy 来读取图像数据。
# importing libraries.
import matplotlib.pyplot as plt
from PIL import Image
# open image using pillow library
image = Image.open("tesla.png")
#show original image
plt.imshow(image)
plt.show()
# grayscale the image
plt.imshow(image.convert("L"), cmap='gray')
plt.show()