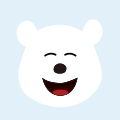
【前端】从零开始:如何轻松将ChatGPT集成到你的Vue项目中!
在当今快速发展的互联网时代,人工智能(AI)已经渗透到我们生活的各个方面。作为前端开发者,如何将强大的ChatGPT功能集成到Vue项目中,成为了许多人关注的焦点。今天,我将带你一步步完成这一过程,让你的应用具备智能对话功能,提升用户体验!✨
一、项目准备:打造坚实的基础
在开始之前,我们需要做好充分的准备工作,确保项目顺利进行。
1. 搭建Vue项目环境
首先,确保你的电脑已经安装了Node.js和npm(Node Package Manager)。如果还没有安装,可以前往Node.js官网下载并安装最新版本。
安装完成后,打开命令行工具,使用以下命令创建一个新的Vue项目:
vue create chatgpt-vue-app
在安装过程中,你会被询问选择项目配置。根据需求选择是否使用TypeScript、路由、状态管理等功能。创建完成后,进入项目目录:
cd chatgpt-vue-app
2. 获取OpenAI API Key
要使用ChatGPT,你需要一个OpenAI的API Key。前往我之前写的CSDN文章查看教程:
【OpenAI】获取OpenAI API Key的多种方式全攻略:从入门到精通,再到详解教程!!:https://blog.csdn.net/zhouzongxin94/article/details/144021130
二、安装依赖:为项目增添动力
在Vue项目中,我们需要安装一些必要的库,以便与ChatGPT进行通信。其中,axios是一个常用的HTTP请求库。
在项目根目录下,运行以下命令安装axios:
npm install axios
安装完成后,项目环境已经准备就绪,可以开始集成ChatGPT了!
三、在Vue组件中调用ChatGPT:实现智能对话
接下来,我们将在Vue项目中创建一个组件,用于与ChatGPT进行交互。
1. 创建ChatGPT组件
在src/components
目录下,新建一个名为ChatGPT.vue
的文件,并添加以下内容:
<template>
<div class="chat-container">
<h2>🤖 ChatGPT 智能助手</h2>
<input v-model="userInput" placeholder="请输入你的问题" />
<button @click="sendQuestion">发送问题</button>
<div v-if="response" class="response">
<strong>ChatGPT:</strong> {{ response }}
</div>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
userInput: '',
response: null,
conversationHistory: []
};
},
methods: {
async sendQuestion() {
if (!this.userInput.trim()) {
alert('请输入有效的问题!');
return;
}
try {
const apiKey = 'YOUR_API_KEY'; // 替换为你自己的OpenAI API Key
const prompt = this.userInput;
// 构建对话消息
let messages = [{"role": "user", "content": prompt}];
if (this.conversationHistory.length > 0) {
messages = this.conversationHistory.concat(messages);
}
// 发送请求到OpenAI API
const response = await axios.post(
'https://api.openai.com/v1/chat/completions',
{
model: "gpt-3.5-turbo",
messages: messages
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
}
);
// 更新对话历史
const assistantMessage = response.data.choices[0].message.content;
this.conversationHistory = messages.concat([{"role": "assistant", "content": assistantMessage}]);
this.response = assistantMessage;
this.userInput = ''; // 清空输入框
} catch (error) {
console.error(error);
this.response = '请求出现错误,请稍后再试。';
}
}
}
};
</script>
<style scoped>
.chat-container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 10px;
background-color: #fafafa;
}
input {
width: 80%;
padding: 10px;
margin-right: 10px;
border-radius: 5px;
border: 1px solid #ccc;
}
button {
padding: 10px 20px;
border: none;
border-radius: 5px;
background-color: #42b983;
color: white;
cursor: pointer;
}
button:hover {
background-color: #369870;
}
.response {
margin-top: 20px;
padding: 10px;
background-color: #e0f7fa;
border-radius: 5px;
}
</style>
2. 解析组件结构
- **模板部分 (
template
)**:
a.一个输入框用于用户输入问题。
b.一个按钮用于发送问题。
c.一个用于展示ChatGPT回复的区域,仅在有回复时显示。
- **脚本部分 (
script
)**:
a.引入axios库,用于发送HTTP请求。
b.定义了userInput
(用户输入)、response
(ChatGPT回复)和conversationHistory
(对话历史)三个数据属性。
c.sendQuestion
方法负责处理用户输入、发送请求并更新对话历史。
- **样式部分 (
style
)**:
a.基本的样式设计,提升用户界面的美观性和用户体验。
3. 在Vue应用中使用ChatGPT组件
打开src/App.vue
文件,引入并使用刚刚创建的ChatGPT
组件:
<template>
<div id="app">
<ChatGPT />
</div>
</template>
<script>
import ChatGPT from './components/ChatGPT.vue';
export default {
name: 'App',
components: {
ChatGPT
}
};
</script>
<style>
/* 可以添加全局样式 */
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
}
</style>
4. 运行项目
一切准备就绪后,返回命令行,运行以下命令启动Vue项目:
npm run serve
打开浏览器,访问http://localhost:8080
(具体端口可能因配置不同而异),即可看到ChatGPT智能助手的界面。输入问题,点击发送,即可与ChatGPT进行对话交流!
四、扩展功能与优化:让应用更上一层楼
集成ChatGPT只是第一步,为了提升用户体验,还可以进行以下优化和功能扩展。
1. 实现多轮对话功能
多轮对话能够让应用与用户进行更自然、更智能的交流。我们已经在组件中添加了conversationHistory
来存储对话历史,但可以进一步优化:
- 保存对话历史:确保对话历史不会因页面刷新而丢失,可以考虑使用本地存储(LocalStorage)来保存。
- 管理对话上下文:限制对话历史的长度,避免发送过长的消息数组导致性能问题。
修改sendQuestion
方法,添加对话历史的管理:
methods: {
async sendQuestion() {
if (!this.userInput.trim()) {
alert('请输入有效的问题!');
return;
}
try {
const apiKey = 'YOUR_API_KEY'; // 替换为你自己的OpenAI API Key
const prompt = this.userInput;
// 从本地存储获取对话历史
let messages = JSON.parse(localStorage.getItem('conversationHistory')) || [];
messages.push({"role": "user", "content": prompt});
// 发送请求到OpenAI API
const response = await axios.post(
'https://api.openai.com/v1/chat/completions',
{
model: "gpt-3.5-turbo",
messages: messages
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
}
);
// 获取ChatGPT回复并更新对话历史
const assistantMessage = response.data.choices[0].message.content;
messages.push({"role": "assistant", "content": assistantMessage});
this.conversationHistory = messages;
this.response = assistantMessage;
this.userInput = ''; // 清空输入框
// 将对话历史保存到本地存储
localStorage.setItem('conversationHistory', JSON.stringify(messages));
} catch (error) {
console.error(error);
this.response = '请求出现错误,请稍后再试。';
}
}
}
2. 添加加载动画与错误提示
为了提升用户体验,可以在请求发送过程中显示加载动画,并在出现错误时给出明确提示。
修改模板部分,添加加载状态和错误提示:
<template>
<div class="chat-container">
<h2>🤖 ChatGPT 智能助手</h2>
<input v-model="userInput" placeholder="请输入你的问题" />
<button @click="sendQuestion" :disabled="isLoading">发送问题</button>
<div v-if="isLoading" class="loading">⏳ 正在生成回答...</div>
<div v-if="response" class="response">
<strong>ChatGPT:</strong> {{ response }}
</div>
<div v-if="error" class="error">{{ error }}</div>
</div>
</template>
在脚本部分,添加isLoading
和error
数据属性,并更新sendQuestion
方法:
data() {
return {
userInput: '',
response: null,
conversationHistory: [],
isLoading: false,
error: null
};
},
methods: {
async sendQuestion() {
if (!this.userInput.trim()) {
alert('请输入有效的问题!');
return;
}
this.isLoading = true;
this.error = null;
try {
const apiKey = 'YOUR_API_KEY'; // 替换为你自己的OpenAI API Key
const prompt = this.userInput;
// 从本地存储获取对话历史
let messages = JSON.parse(localStorage.getItem('conversationHistory')) || [];
messages.push({"role": "user", "content": prompt});
// 发送请求到OpenAI API
const response = await axios.post(
'https://api.openai.com/v1/chat/completions',
{
model: "gpt-3.5-turbo",
messages: messages
},
{
headers: {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
}
}
);
// 获取ChatGPT回复并更新对话历史
const assistantMessage = response.data.choices[0].message.content;
messages.push({"role": "assistant", "content": assistantMessage});
this.conversationHistory = messages;
this.response = assistantMessage;
this.userInput = ''; // 清空输入框
// 将对话历史保存到本地存储
localStorage.setItem('conversationHistory', JSON.stringify(messages));
} catch (error) {
console.error(error);
this.error = '请求出现错误,请稍后再试。';
} finally {
this.isLoading = false;
}
}
}
在样式部分,添加加载和错误提示的样式:
.loading {
margin-top: 20px;
color: #ff9800;
}
.error {
margin-top: 20px;
color: #f44336;
}
3. 添加清除对话历史功能
为了让用户能够清除对话历史,提升体验,可以添加一个“清除聊天”按钮。
在模板部分,添加按钮:
<button @click="clearHistory" :disabled="isLoading">清除聊天</button>
在脚本部分,添加clearHistory
方法:
methods: {
// ...已有方法
clearHistory() {
this.conversationHistory = [];
localStorage.removeItem('conversationHistory');
this.response = null;
this.userInput = '';
}
}
并在样式中调整按钮布局:
button {
margin-top: 10px;
padding: 10px 20px;
/* 其他样式保持不变 */
}
button + button {
margin-left: 10px;
background-color: #f44336;
}
button + button:hover {
background-color: #d32f2f;
}
4. 输入验证与限制
为了避免用户输入过长或不合法的内容,可以在发送问题前进行验证和限制。
修改sendQuestion
方法,添加输入长度限制:
methods: {
async sendQuestion() {
const trimmedInput = this.userInput.trim();
if (!trimmedInput) {
alert('请输入有效的问题!');
return;
}
if (trimmedInput.length > 500) {
alert('问题太长,请限制在500字以内。');
return;
}
// 继续执行发送问题的逻辑
// ...
},
// 其他方法保持不变
}
五、提升应用性能与用户体验
在完成基本功能后,我们还可以通过以下方式进一步优化应用。
1. 优化API调用
为了避免频繁调用API,可以设置防抖(debounce)机制,限制用户发送问题的频率。例如,用户在短时间内频繁点击发送按钮时,仅保留最后一次点击。
可以使用lodash库中的debounce
函数实现:
npm install lodash
在组件中引入并应用:
import { debounce } from 'lodash';
export default {
// ...已有内容
created() {
this.sendQuestion = debounce(this.sendQuestion, 1000);
},
// ...其他内容
}
这样,每次调用sendQuestion
方法时,会有1秒的间隔,防止过于频繁的请求。
2. 响应式设计
确保应用在不同设备上都有良好的显示效果,采用响应式设计。
在样式部分,添加媒体查询:
.chat-container {
max-width: 600px;
margin: 50px auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 10px;
background-color: #fafafa;
}
@media (max-width: 768px) {
.chat-container {
margin: 20px;
padding: 15px;
}
input {
width: 100%;
margin-bottom: 10px;
}
button {
width: 100%;
margin-bottom: 10px;
}
}
3. 美化界面
通过进一步美化界面,使应用更加吸引人。可以使用CSS框架如Bootstrap或Element UI,也可以自定义样式。
例如,使用渐变背景和卡片式设计:
.chat-container {
max-width: 600px;
margin: 50px auto;
padding: 30px;
border-radius: 15px;
background: linear-gradient(135deg, #f0f4f8, #d9e2ec);
box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1);
}
h2 {
text-align: center;
margin-bottom: 20px;
color: #333;
}
.input-group {
display: flex;
flex-direction: column;
}
input {
padding: 12px;
border-radius: 8px;
border: 1px solid #ccc;
margin-bottom: 10px;
font-size: 16px;
}
button {
padding: 12px;
border: none;
border-radius: 8px;
background-color: #42b983;
color: white;
font-size: 16px;
cursor: pointer;
transition: background-color 0.3s;
}
button:hover {
background-color: #369870;
}
.response, .loading, .error {
margin-top: 20px;
padding: 15px;
border-radius: 8px;
font-size: 16px;
}
.response {
background-color: #e0f7fa;
}
.loading {
color: #ff9800;
}
.error {
background-color: #ffebee;
color: #f44336;
}
💥 VSvode-大模型AI工具👇🏻🌟🌟 -【CodeMoss】集成了13种GPT大模型(包含GPT4、o1等)、提示词助手100+、支持Open API调用、自定义助手、文件上传等强大功能,助您提升工作效率!
六、总结
通过以上步骤,我们成功将ChatGPT集成到了Vue项目中,实现了一个功能完备的智能对话应用。从项目准备、依赖安装,到组件开发、功能扩展,再到性能优化和用户体验提升,每一步都为最终的成果打下了坚实的基础。
本文转载自爱学习的蝌蚪,作者: hpstream
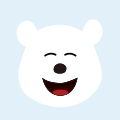